SQL is a potent programming language that plays a significant part in modern society. We can exchange data and interface with relational databases using it. SQL is used everywhere there is data. This encompasses governmental bodies, non-profit institutions, and enterprises of all kinds operating in every sector, from shipping to shoe sales. Additionally, one of the most sought-after technical talents for data professions is SQL.
As you may have heard, data has surpassed even fossil fuels like oil in value and is currently the most valuable commodity in the world. So how can we make the most of this resource to acquire knowledge and make astute decisions?
What is SQL?
SQL, pronounced “sequel” or spelled out as “S-Q-L,” is a domain-specific language used for managing and manipulating relational databases. Developed in the 1970s by IBM researchers Donald D. Chamberlin and Raymond F. Boyce, SQL was designed to be an intuitive language that could allow users to interact with large datasets. It became an ANSI (American National Standards Institute) standard in 1986, ensuring that it remains a universal language for database management systems (DBMS).
The Fundamentals of SQL
SQL’s power lies in its simplicity and versatility. At its core, SQL consists of several key operations that can be broadly categorized into:
- Data Query Language (DQL): The most common SQL operation is querying data. The
SELECT
statement is used to retrieve data from one or more tables. With the ability to filter, sort, and aggregate data, SQL allows users to derive meaningful insights from raw data.sqlCopy codeSELECT first_name, last_name FROM employees WHERE department = 'Sales';
- Data Definition Language (DDL): DDL commands define and modify the structure of database objects, such as tables and indexes. Common DDL statements include
CREATE
,ALTER
, andDROP
.sqlCopy codeCREATE TABLE employees ( employee_id INT PRIMARY KEY, first_name VARCHAR(50), last_name VARCHAR(50), department VARCHAR(50) );
- Data Manipulation Language (DML): DML commands allow users to insert, update, delete, and manipulate data within tables. The primary DML statements are
INSERT
,UPDATE
, andDELETE
.sqlCopy codeINSERT INTO employees (employee_id, first_name, last_name, department) VALUES (1, 'John', 'Doe', 'Sales');
- Data Control Language (DCL): DCL commands control access to data within the database. The main DCL statements are
GRANT
andREVOKE
, which are used to assign and remove permissions.sqlCopy codeGRANT SELECT ON employees TO user1;
- Transaction Control Language (TCL): TCL commands manage transactions within the database, ensuring data integrity. The key TCL statements are
COMMIT
,ROLLBACK
, andSAVEPOINT
.sqlCopy codeBEGIN; UPDATE employees SET department = 'Marketing' WHERE employee_id = 1; COMMIT;
The Importance of SQL in Data Management
SQL is the backbone of modern data management systems for several reasons:
1. Efficiency and Performance
SQL is optimized for interacting with large datasets, allowing users to quickly retrieve and manipulate data. Its ability to perform complex queries and aggregate data efficiently makes it indispensable for businesses that need to process vast amounts of information.
2. Standardization
As an ANSI standard language, SQL provides a consistent way to interact with different database systems, such as MySQL, PostgreSQL, Oracle, and Microsoft SQL Server. This standardization simplifies learning and using SQL, enabling database administrators and developers to transition between systems with ease.
3. Data Integrity and Security
SQL offers robust features for maintaining data integrity and security. Constraints such as primary keys, foreign keys, and unique keys ensure that data remains consistent and accurate. Additionally, SQL’s DCL commands provide granular control over user access, helping to protect sensitive data.
4. Versatility and Flexibility
SQL is versatile enough to handle various tasks, from simple data retrieval to complex data transformations. Its ability to integrate with other programming languages and tools, such as Python, R, and Excel, makes SQL a crucial component in data analytics and reporting workflows.
SQL in Action: Real-World Applications
SQL’s versatility extends to numerous real-world applications across various industries:
1. Business Intelligence and Analytics
SQL is a fundamental tool in business intelligence (BI) and analytics platforms. Analysts use SQL to extract data from databases, transform it into meaningful insights, and visualize trends and patterns. BI tools like Tableau, Power BI, and Looker often rely on SQL queries to power their dashboards and reports.
2. Web Development
In web development, SQL is used to interact with databases that store user data, product information, and transaction records. SQL enables developers to create dynamic, data-driven web applications that can efficiently handle user interactions and requests.
3. Finance and Banking
The finance industry relies heavily on SQL for managing transaction records, customer data, and financial reports. SQL’s ability to handle complex queries and ensure data accuracy makes it a crucial tool for risk assessment, fraud detection, and regulatory compliance.
4. Healthcare
Healthcare organizations use SQL to manage patient records, track treatment outcomes, and analyze clinical data. SQL enables healthcare providers to access critical information quickly, improving patient care and operational efficiency.
5. E-commerce
E-commerce platforms leverage SQL to manage product catalogs, customer orders, and inventory data. SQL allows businesses to analyze sales trends, personalize customer experiences, and optimize supply chain operations.
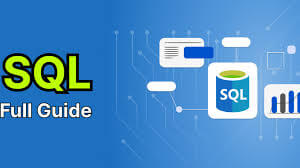
Advanced SQL Concepts
Beyond the basics, SQL offers advanced features that enable users to perform sophisticated data operations:
1. Joins and Subqueries
Joins allow users to combine data from multiple tables based on related columns. Common types of joins include INNER JOIN
, LEFT JOIN
, RIGHT JOIN
, and FULL JOIN
.
sqlCopy codeSELECT employees.first_name, employees.last_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
Subqueries, or nested queries, allow users to perform operations within a larger query, enabling complex data retrieval.
sqlCopy codeSELECT first_name, last_name
FROM employees
WHERE department_id = (
SELECT department_id
FROM departments
WHERE department_name = 'Sales'
);
2. Indexes
Indexes improve query performance by allowing the database to quickly locate specific rows. While indexes enhance read operations, they can impact write performance, so careful consideration is needed when designing them.
sqlCopy codeCREATE INDEX idx_department ON employees (department_id);
3. Stored Procedures and Functions
Stored procedures and functions are precompiled SQL code that can be executed with a single call. They help improve performance and ensure consistent data processing.
sqlCopy codeCREATE PROCEDURE GetEmployeeByDepartment (IN dept_id INT)
BEGIN
SELECT first_name, last_name
FROM employees
WHERE department_id = dept_id;
END;
4. Triggers
Triggers are automatic actions executed in response to specific events on a table, such as INSERT
, UPDATE
, or DELETE
. They are useful for enforcing business rules and maintaining data integrity.
sqlCopy codeCREATE TRIGGER before_employee_insert
BEFORE INSERT ON employees
FOR EACH ROW
BEGIN
SET NEW.created_at = NOW();
END;
5. Transactions
Transactions group multiple SQL operations into a single unit of work, ensuring that either all operations succeed or none at all. This is essential for maintaining data consistency in applications that require atomic operations.
sqlCopy codeBEGIN;
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
UPDATE accounts SET balance = balance + 100 WHERE account_id = 2;
COMMIT;
Best Practices for Writing SQL Queries
Writing efficient and maintainable SQL queries is crucial for database performance and reliability. Here are some best practices to follow:
1. Use Descriptive Names
Use meaningful names for tables, columns, and aliases to improve query readability and maintainability.
sqlCopy codeSELECT e.first_name, e.last_name, d.department_name
FROM employees AS e
JOIN departments AS d ON e.department_id = d.department_id;
2. Optimize Joins
Minimize the number of joins and use the appropriate join type to reduce query complexity and improve performance.
3. Filter Early
Apply filters as early as possible in the query to reduce the amount of data processed and improve performance.
sqlCopy codeSELECT first_name, last_name
FROM employees
WHERE department_id = 1
AND hire_date >= '2024-01-01';
4. Avoid Using SELECT *
Specify only the necessary columns in your queries to reduce the amount of data retrieved and improve performance.
sqlCopy codeSELECT first_name, last_name, department
FROM employees;
5. Use Indexes Wisely
Create indexes on columns that are frequently used in search conditions and joins, but avoid over-indexing, which can impact write performance.
6. Normalize Data
Design your database using normalization principles to reduce redundancy and improve data integrity. However, consider denormalization for read-heavy applications to enhance performance.
The Future of SQL
Despite the emergence of NoSQL databases and new data management technologies, SQL remains a vital skill and tool in the data landscape. As data volumes continue to grow, SQL is evolving to meet new challenges, with innovations such as:
1. SQL on Big Data
SQL engines like Apache Hive and Google BigQuery allow users to run SQL queries on massive datasets stored in distributed file systems, bridging the gap between traditional databases and big data platforms.
2. Cloud-Based SQL Services
Cloud providers offer managed SQL databases, such as Amazon RDS, Google Cloud SQL, and Azure SQL Database, simplifying database management and scaling for organizations of all sizes.
3. Graph and NoSQL Databases with SQL Interfaces
Some NoSQL and graph databases, such as Amazon Neptune and Azure Cosmos DB, provide SQL-like query languages, making it easier for developers to leverage SQL skills in non-relational data models.
4. AI and Machine Learning Integration
SQL is increasingly integrated with AI and machine learning platforms, enabling data scientists to perform advanced analytics and predictive modeling directly within SQL environments.
Conclusion
SQL is an indispensable tool in the modern data landscape, enabling organizations to harness the power of their data for informed decision-making and competitive advantage. Its simplicity, efficiency, and versatility make it a must-learn language for anyone involved in data management, analytics, or application development. As technology continues to evolve, SQL’s adaptability ensures that it will remain a crucial skill for years to come.
Whether you’re a seasoned database administrator or a novice data enthusiast, mastering SQL opens up a world of possibilities in the realm of data-driven innovation.